【Java面试】MyBatis是如何进行分页的?
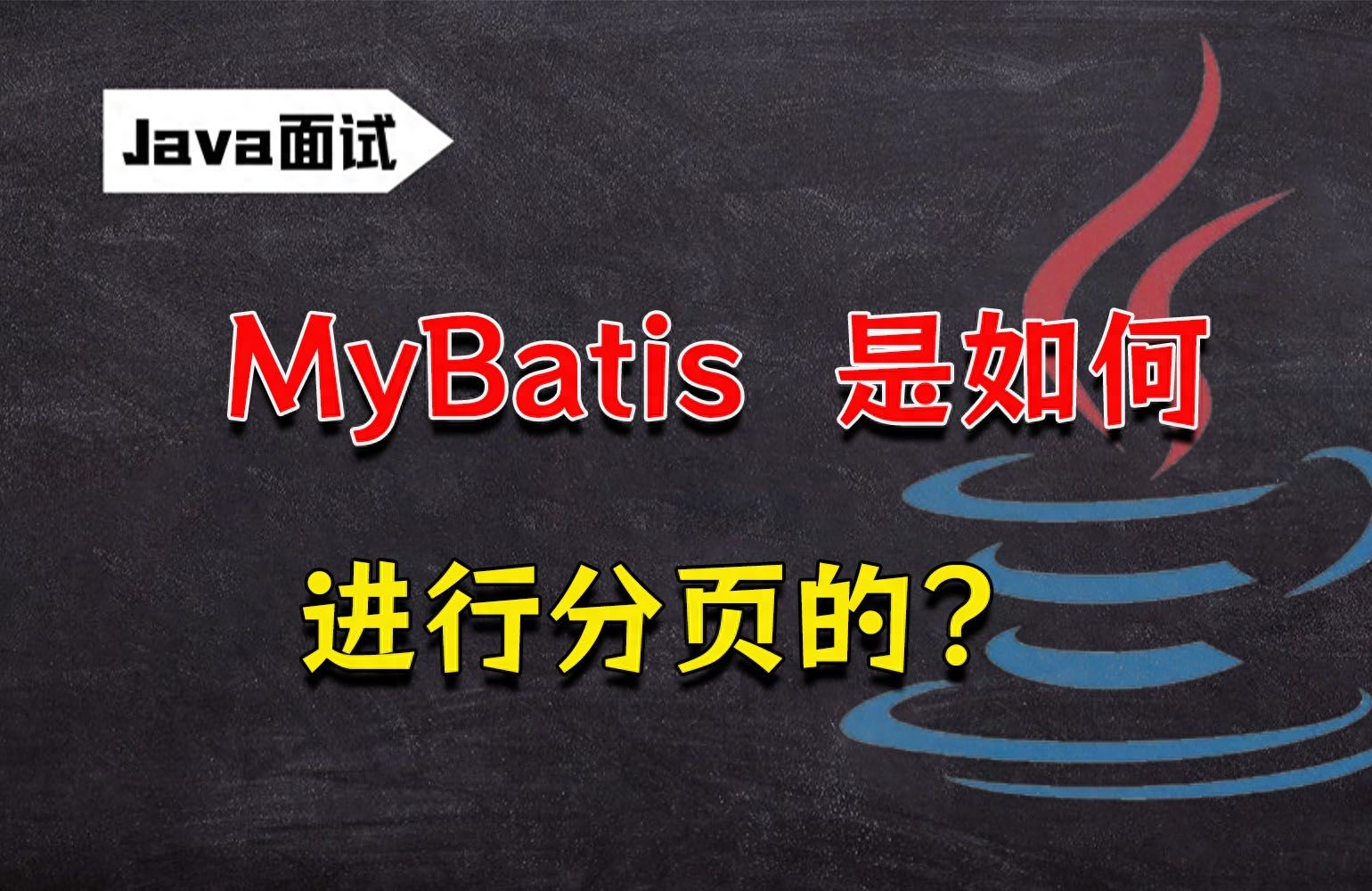
基于 SQL 语句的分页方式
使用数据库原生的 SQL 语句:不同的数据库有自己的分页查询语法。例如,在 MySQL 中,可以使用LIMIT关键字进行分页。假设我们有一个用户表users,要查询第pageNo页,每页显示pageSize条记录,查询语句可以写成SELECT * FROM users LIMIT (pageNo - 1) * pageSize, pageSize。在 MyBatis 的mapper.xml文件中,定义如下查询方法:
<select id="selectUsersByPage" resultMap="UserResultMap">
SELECT * FROM users LIMIT #{offset}, #{limit}
</select>
其中#{offset}和#{limit}是传入的参数,分别代表偏移量((pageNo - 1) * pageSize)和每页显示的记录数。在对应的 Java 接口方法中,传入正确的参数值即可实现分页查询。这种方式简单直接,性能较好,但缺点是不同数据库的分页语法不同,当数据库切换时,需要修改 SQL 语句。
- 使用数据库存储过程进行分页:对于一些复杂的分页场景,或者为了提高性能,可以使用存储过程来实现分页。以 Oracle 数据库为例,可以创建一个存储过程来接受页码和每页记录数作为参数,然后在存储过程内部进行分页查询。在 MyBatis 中,可以通过{call procedure_name(#{param1}, #{param2})}的方式调用存储过程进行分页。不过使用存储过程会增加数据库的维护成本,并且不同数据库的存储过程语法差异较大。
使用 MyBatis 插件实现分页
- 原理:MyBatis 插件是一种拦截机制,可以在 MyBatis 执行 SQL 语句的过程中进行拦截和修改。对于分页插件,它通常会拦截Executor的query方法,在查询之前对 SQL 语句进行修改,添加分页相关的语法,然后再执行修改后的 SQL 语句。
- 常见的分页插件(以 PageHelper 为例):
- 配置插件:首先在项目的依赖中添加PageHelper的依赖,然后在 MyBatis 的配置文件(mybatis - config.xml)中进行插件配置,如下所示:
<plugins>
<plugin interceptor="com.github.pagehelper.PageInterceptor">
<property name="helperDialect" value="mysql"/>
<property name="reasonable" value="true"/>
</plugin>
</plugins>
这里配置了PageInterceptor作为分页插件,并且指定了数据库方言(如mysql)和一些其他属性(如reasonable表示是否开启合理化分页,当页码小于 1 或超过最大页码时进行修正)。
- 使用插件进行分页:在业务代码中,使用非常方便。例如,在一个服务类中,查询用户列表并分页:
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class UserService {
private UserMapper userMapper;
public PageInfo<User> getUsersByPage(int pageNo, int pageSize) {
// 开启分页
PageHelper.startPage(pageNo, pageSize);
List<User> userList = userMapper.selectUsers();
return new PageInfo<>(userList);
}
}
在这个例子中,通过PageHelper.startPage(pageNo, pageSize)开启分页,然后执行查询操作,PageHelper会自动拦截查询语句并添加分页语法,最后将查询结果封装到PageInfo对象中,PageInfo对象包含了分页相关的信息,如总记录数、总页数、当前页码等。
自定义拦截器实现分页
- 拦截器实现步骤:实现Interceptor接口:创建一个自定义的拦截器类,实现org.apache.ibatis.plugin.Interceptor接口。这个接口有三个方法需要实现,分别是intercept、plugin和setProperties。在intercept方法中,实现对 SQL 语句的拦截和修改逻辑,用于添加分页语法。
- 示例代码:
import org.apache.ibatis.executor.Executor;
import org.apache.ibatis.mapping.BoundSql;
import org.apache.ibatis.mapping.MappedStatement;
import org.apache.ibatis.plugin.Interceptor;
import org.apache.ibatis.plugin.Intercepts;
import org.apache.ibatis.plugin.Invocation;
import org.apache.ibatis.plugin.Signature;
import org.apache.ibatis.session.ResultHandler;
import org.apache.ibatis.session.RowBounds;
import java.util.Properties;
@Intercepts({
@Signature(type = Executor.class, method = "query", args = {MappedStatement.class, Object.class, RowBounds.class, ResultHandler.class})
})
public class CustomPaginationInterceptor implements Interceptor {
@Override
public Object intercept(Invocation invocation) throws Throwable {
// 获取原始的参数
Object[] args = invocation.getArgs();
MappedStatement mappedStatement = (MappedStatement) args[0];
Object parameter = args[1];
RowBounds rowBounds = (RowBounds) args[2];
ResultHandler resultHandler = (ResultHandler) args[3];
// 判断是否是查询操作并且有分页参数
if (rowBounds!= RowBounds.DEFAULT &&!rowBounds.equals(RowBounds.NO_ROW_LIMIT)) {
// 获取原始的 BoundSql
BoundSql boundSql = mappedStatement.getBoundSql(parameter);
String originalSql = boundSql.getSql();
// 计算偏移量和每页条数
int offset = rowBounds.getOffset();
int limit = rowBounds.getLimit();
// 根据数据库类型构造分页 SQL
String databaseType = mappedStatement.getConfiguration().getDatabaseId();
String paginatedSql;
if ("mysql".equalsIgnoreCase(databaseType)) {
paginatedSql = originalSql + " LIMIT " + offset + "," + limit;
} else {
// 其他数据库可以根据情况进行处理
paginatedSql = originalSql;
}
// 创建新的 BoundSql 对象
BoundSql newBoundSql = new BoundSql(mappedStatement.getConfiguration(), paginatedSql, boundSql.getParameterMappings(), parameter);
// 创建新的 MappedStatement 对象
MappedStatement newMappedStatement = new MappedStatement.Builder(mappedStatement.getConfiguration(), mappedStatement.getId(), newBoundSql, mappedStatement.getSqlCommandType()).build();
// 修改参数中的 MappedStatement
args[0] = newMappedStatement;
}
// 执行原始的查询方法
return invocation.proceed();
}
@Override
public Object plugin(Object target) {
return Interceptor.super.plugin(target);
}
@Override
public void setProperties(Properties properties) {
Interceptor.super.setProperties(properties);
}
}
上述代码中,CustomPaginationInterceptor实现了Interceptor接口,在intercept方法中,首先获取传入的参数,判断是否有分页参数,如果有,则根据数据库类型构造分页 SQL,然后创建新的BoundSql和MappedStatement对象,最后修改参数并执行原始的查询方法。
- 配置拦截器:和配置分页插件类似,在 MyBatis 的配置文件(mybatis - config.xml)中添加自定义拦截器的配置,让 MyBatis 能够识别并加载拦截器。
<plugins>
<plugin interceptor="com.yourpackage.CustomPaginationInterceptor"/>
</plugins>
使用 RowBounds 实现逻辑分页
- RowBounds 原理:RowBounds是 MyBatis 提供的一种逻辑分页方式。它通过在查询时限制返回的结果集范围来实现分页效果。在底层,MyBatis 会根据RowBounds参数从数据库中查询所有符合条件的记录,然后在内存中对结果集进行截取,只返回指定范围内的记录。例如,设置RowBounds为从第 10 条记录开始,取 20 条记录,MyBatis 会先查询出所有符合条件的记录,然后在内存中选取第 10 到第 29 条记录返回。
- 适用场景:
- 数据量较小的情况:当查询的数据量相对较小,且不会对内存造成较大压力时,RowBounds是一种简单有效的分页方式。例如,对于一些配置表或者小型数据集的查询,使用RowBounds可以快速实现分页功能,而不需要复杂的 SQL 语句修改或者插件配置。
- 不依赖数据库特性的场景:如果应用需要在不同的数据库环境中运行,且不想使用数据库特定的分页语法,RowBounds是一个不错的选择。因为它是基于 MyBatis 内部的逻辑实现分页,不依赖于像 MySQL 的LIMIT或者 Oracle 的ROWNUM等数据库特有的分页功能,这样可以提高代码在不同数据库之间的移植性。
- 快速原型开发和简单应用场景:在开发初期或者一些对分页功能要求不高的简单应用中,RowBounds可以快速实现分页效果。开发人员可以先使用RowBounds实现基本的分页功能,随着应用的发展和对性能要求的提高,再考虑切换到更高效的分页方式,如基于 SQL 语句或者分页插件的方式。
- 使用示例:在 Java 代码中,可以在调用 MyBatis 的Mapper方法时传入RowBounds对象来实现分页。例如:
import org.apache.ibatis.session.RowBounds;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class UserService {
private UserMapper userMapper;
public List<User> getUsersByPage(int pageNo, int pageSize) {
int offset = (pageNo - 1) * pageSize;
RowBounds rowBounds = new RowBounds(offset, pageSize);
return userMapper.selectUsers(rowBounds);
}
}
在这个例子中,首先根据页码和每页记录数计算出偏移量,然后创建RowBounds对象并传入Mapper方法。在Mapper接口中,定义方法如下:
List<User> selectUsers(RowBounds rowBounds);
MyBatis 会在执行这个方法时,根据传入的RowBounds参数进行逻辑分页。这种方式的优点是简单易用,不需要修改 SQL 语句,也不需要依赖数据库的特定分页语法。但是,当查询的数据量非常大时,将所有符合条件的记录都查询出来再在内存中进行截取会消耗大量内存,性能可能会受到很大影响。